APCS程式檢測 -實作題1051029 第3題 定時K彈
本題會使用到 C++ 的 STL vector,如果不熟悉的話可以來這裡看看
https://husking-studio.com/cpp-stl-vector/
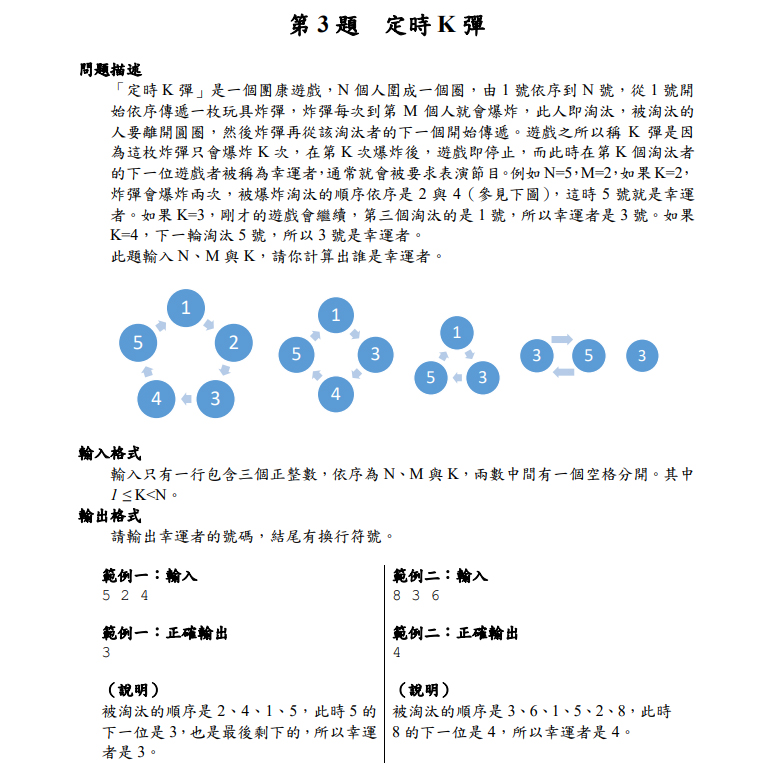

找 vecPlayer 裡找爆炸玩家
程式碼
//找會爆炸的索引值
//nVecSize - STL vector 裡的資料總數
//nStartIndex - 從哪個玩家開始,注意這是索引值(index),不是玩家編號
//nStep - 向下找幾個
int GetExplodeIndex(int nVecSize, int nStartIndex, int nStep)
{
int nExplodeIndex = nStartIndex;
for (int i = 0; i < (nStep-1); i++)
{
nExplodeIndex = nExplodeIndex + 1;
if (nExplodeIndex >= nVecSize) //找到最後,再從頭開始
nExplodeIndex = 0;
}
return nExplodeIndex;
}
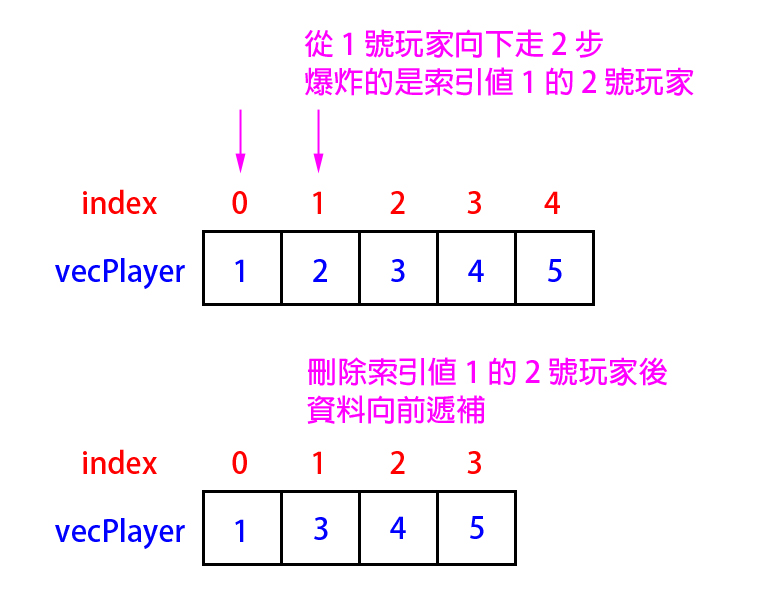
完整程式碼
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using namespace std;
int GetExplodeIndex(int nVecSize, int nStartIndex, int nStep);
vector<int>::iterator GetVectorItByIndex(vector<int>* pVector, int nIndex);
int main()
{
int N = 0;
int M = 0;
int K = 0;
//處理輸入
string strInput;
getline(cin, strInput);
stringstream delim(strInput);
string pch;
getline(delim, pch, ' ');
N = stoi(pch);
getline(delim, pch, ' ');
M = stoi(pch);
getline(delim, pch, ' ');
K = stoi(pch);
//設定玩家 vector
vector<int> vecPlayer;
for (int i = 0; i < N; i++)
vecPlayer.push_back(i + 1);
int nStartIndex = 0; //開始的索引值
int nExplodeIndex = 0; //會爆炸的索引值
int nLuckyIndex = 0; //爆炸的下一個索引值
vector<int>::iterator eraseIt;
for (int i = 0; i < K; i++)
{
nExplodeIndex = GetExplodeIndex((int)vecPlayer.size(), nStartIndex, M); //找會爆炸的索引值
//cout << "Explode player:" << vecPlayer[nExplodeIndex] << endl; //可以印出爆炸玩家
eraseIt = GetVectorItByIndex(&vecPlayer, nExplodeIndex); //找會爆炸的索引值的 it
vecPlayer.erase(eraseIt); //將會爆炸的索引值的 it 從玩家 vector 中刪除
nStartIndex = nExplodeIndex; //下一輪從爆炸這裡開始
nLuckyIndex = nExplodeIndex + 1; //爆炸的下一個索引值,就是幸運玩家的索引值
if (nLuckyIndex >= (int)vecPlayer.size())
nLuckyIndex = 0;
}
cout << vecPlayer[nLuckyIndex] << endl;
system("pause");
return 0;
}
vector<int>::iterator GetVectorItByIndex(vector<int>* pVector, int nIndex)
{
if (nIndex < 0 || nIndex >= (int)pVector->size())
return pVector->end();
vector<int>::iterator it;
it = pVector->begin();
for (int i = 0; i < nIndex; i++)
it++;
return it;
}
int GetExplodeIndex(int nVecSize, int nStartIndex, int nStep)
{
int nExplodeIndex = nStartIndex;
for (int i = 0; i < (nStep - 1); i++)
{
nExplodeIndex = nExplodeIndex + 1;
if (nExplodeIndex >= nVecSize)
nExplodeIndex = 0;
}
return nExplodeIndex;
}

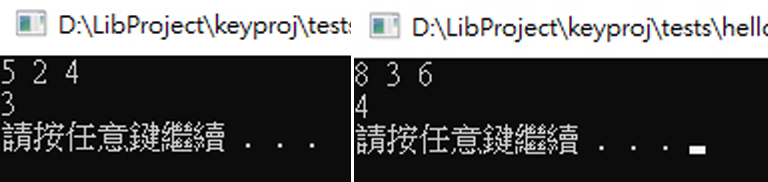