Lua 與 C 擴充-簡單函式
環境
windows 11 64bit
Visual Studio 2022
Lua 5.4.6
C擴充程式碼 – mytestlib.c
#include <K:\Lua\546\lib17\include\lua.h>
#include <K:\Lua\546\lib17\include\lualib.h>
#include <K:\Lua\546\lib17\include\lauxlib.h>
#include <K:\Lua\546\lib17\include\luaconf.h>
#include <string.h>
#pragma comment(lib,"K:\\Lua\\546\\lib17\\x86\\Release\\lua54.lib")
static int luaAdd(lua_State* L)
{
double p1 = luaL_checknumber(L, 1);
double p2 = luaL_checknumber(L, 2);
lua_pushnumber(L, p1 + p2);
return 1;
}
static int luaSub(lua_State* L)
{
double p1 = luaL_checknumber(L, 1);
double p2 = luaL_checknumber(L, 2);
lua_pushnumber(L, p1 - p2);
return 1;
}
static int luaHelloString(lua_State* L)
{
const char* Name = luaL_checkstring(L, 1);
char sz[128] = "Hello!";
strcat_s(sz, sizeof(sz), Name);
lua_pushstring(L, sz);
return 1;
}
static const luaL_Reg mylibs[] =
{
{"add", luaAdd},
{"sub", luaSub},
{"hello", luaHelloString},
{NULL, NULL}
};
int __declspec(dllexport) luaopen_mytestlib(lua_State * L)
{
lua_newtable(L);
luaL_setfuncs(L, mylibs, 0);
return 1;
}
1. 最簡單的方式
寫compile指令 – compile.bat
cl /LD /MD mytestlib.c
打開VS的文字控制編譯器 – VC.bat
@echo off
%comspec% /k ""C:\Program Files\Microsoft Visual Studio\2022\Community\VC\Auxiliary\Build\vcvarsall.bat"" x86
打開之後輸入 compile,編譯完成

主要是要產生 mytestlib.dll

test.lua
local mytest = require "mytestlib"
print (mytest.add(1.0,2.0))
print (mytest.sub(1.0,2.0))
print (mytest.hello("Charlie"))
把 mytestlib.dll 和 test.lua 放一起,執行 test.lua 後的結果
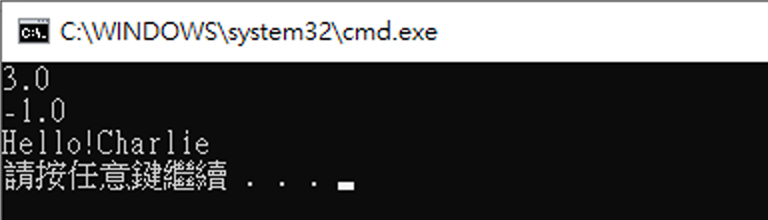
2. 用 Visual Studio 2022 產生 mytestlib.dll


這會是 dll 檔的檔名

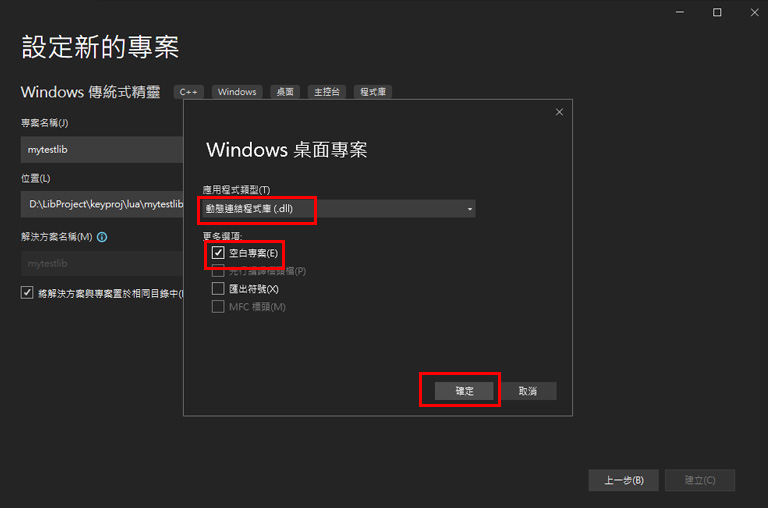
把剛剛的 mytestlib.c 放進來

不過要修改一下引用的 lib
#ifdef _WIN64
#ifdef _DEBUG
#pragma comment(lib,"K:\\Lua\\546\\lib17\\x64\\Debug\\lua54.lib")
#else
#pragma comment(lib,"K:\\Lua\\546\\lib17\\x64\\Release\\lua54.lib")
#endif
#else
#ifdef _DEBUG
#pragma comment(lib,"K:\\Lua\\546\\lib17\\x86\\Debug\\lua54.lib")
#else
#pragma comment(lib,"K:\\Lua\\546\\lib17\\x86\\Release\\lua54.lib")
#endif
#endif
建置成功之後也是能產生 mytestlib.dll
3. C++
mytestlib.cpp
#include <K:\Lua\546\lib17\include\lua.hpp>
#include <string.h>
#pragma comment(lib,"K:\\Lua\\546\\lib17\\x86\\Release\\lua54.lib")
static int luaAdd(lua_State* L)
{
double p1 = luaL_checknumber(L, 1);
double p2 = luaL_checknumber(L, 2);
lua_pushnumber(L, p1 + p2);
return 1;
}
static int luaSub(lua_State* L)
{
double p1 = luaL_checknumber(L, 1);
double p2 = luaL_checknumber(L, 2);
lua_pushnumber(L, p1 - p2);
return 1;
}
static int luaHelloString(lua_State* L)
{
const char* Name = luaL_checkstring(L, 1);
char sz[128] = "Hello!";
strcat_s(sz, sizeof(sz), Name);
lua_pushstring(L, sz);
return 1;
}
static const luaL_Reg mylibs[] =
{
{"add", luaAdd},
{"sub", luaSub},
{"hello", luaHelloString},
{NULL, NULL}
};
extern "C"
{
int __declspec(dllexport) luaopen_mytestlib(lua_State * L)
{
lua_newtable(L);
luaL_setfuncs(L, mylibs, 0);
return 1;
}
}
寫compile指令 – compile.bat
cl /LD /MD mytestlib.cpp
之後的步驟跟前面C的都一樣。
Lua5.2 之後,取消掉了 luaL_register,關於這部分造成的問題,可以參考最下面的網址。
回到目錄
https://husking-studio.com/extending-lua-with-c
參考網址
https://iter01.com/554581.html
http://www.yjxsoft.com/forum.php?mod=viewthread&tid=4384
https://www.136.la/net/show-9708.html