Unity 內建的 UGUI 中,最基本的就是 Text,常常要用來印資訊,這篇文章會介紹一些基本用法。
環境
Unity 2021.1.10f1 (64-bit)
先簡單設定一個場景
只有一個 text 和一個 button
改 text 的名字、顏色和大小
其他都維持預設
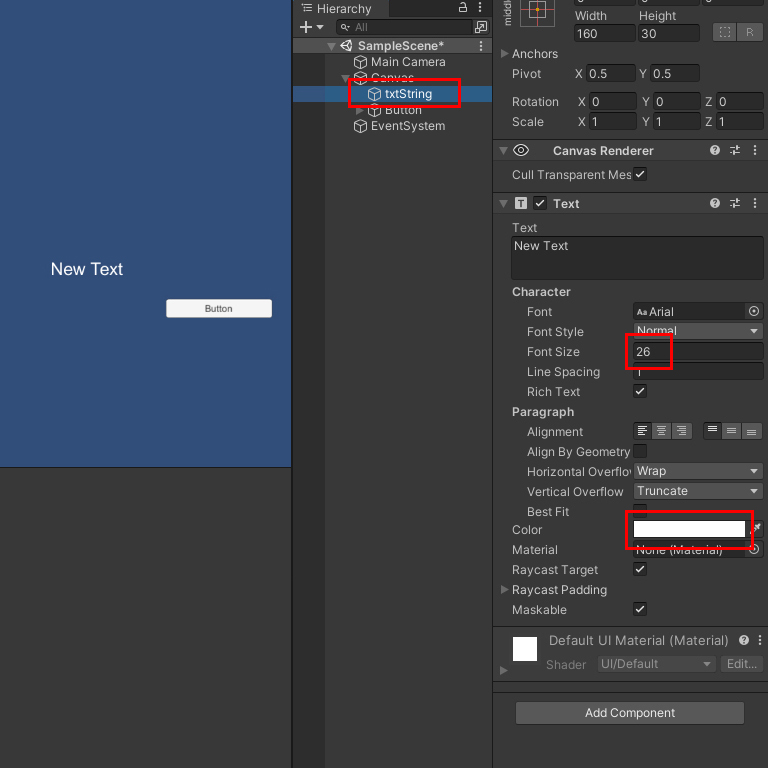
然後寫程式碼去修改內容
測試程式碼1 ButtonChange.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ButtonChange : MonoBehaviour
{
void Start()
{
}
void Update()
{
}
public void OnButtonClick()
{
string str = "The only way Ive found to do this has been to add the elements. Then wait one frame, then disable the verticalLayoutGroup and contentSizeFitter.";
GameObject goText = GameObject.Find("txtString");
Text t = goText.GetComponent<Text>();
t.text = str;
}
}
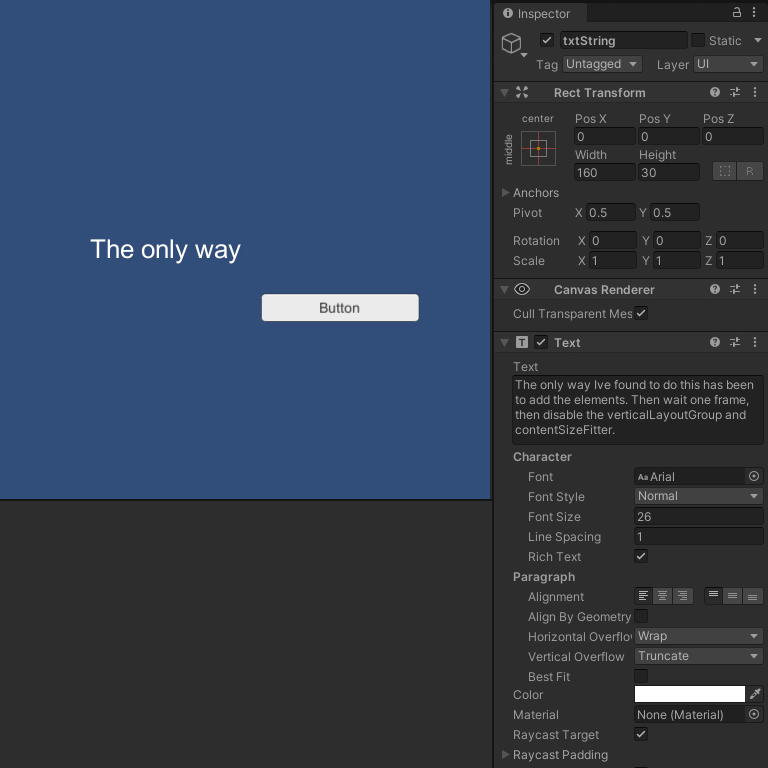
因為字太長所以會被切掉
當然調整 text 的 width 也是可以
測試程式碼2 ButtonChange.cs
public void OnButtonClick()
{
//string str = "abcdefghijklmnop";
string str = "llllllllllllllll";
GameObject goText = GameObject.Find("txtString");
RectTransform rcText = goText.GetComponent<RectTransform>();
int nStrLen = str.Length;
rcText.sizeDelta = new Vector2(nStrLen * 13, rcText.sizeDelta.y);
Text t = goText.GetComponent<Text>();
t.text = str;
}
但是會出現一個問題
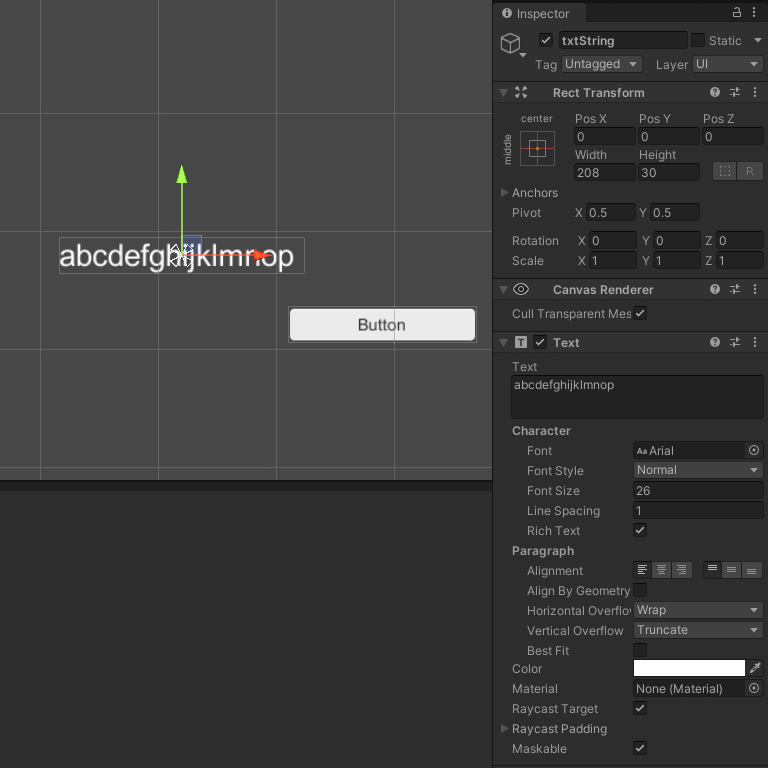
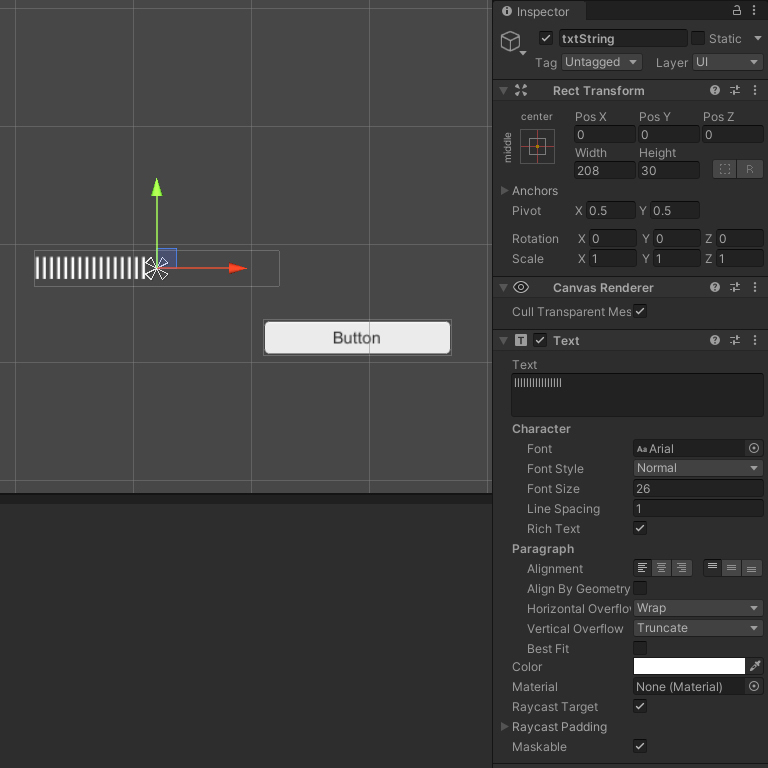
明明是一樣的字數
但看起來怎麼不一樣?
原因就在於很多英文字型其實是不等寬的
所以用字串的字數去設定 text 的 width 是不準的
尤其如果還中英文夾雜
那會連字串的字數都很難計算
那要怎麼辦呢?
在 text 元件加入 component -> Content Size Fitter
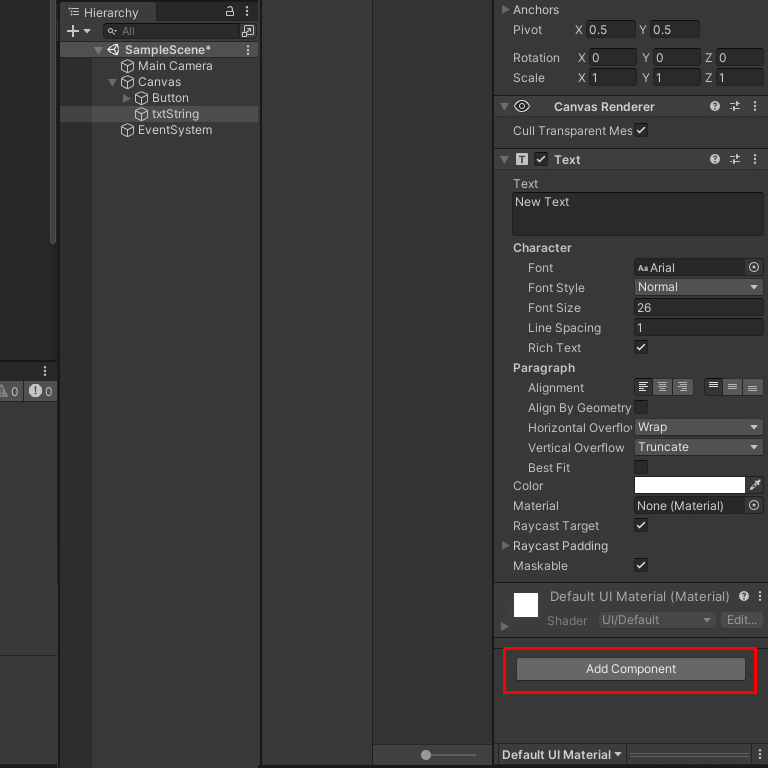
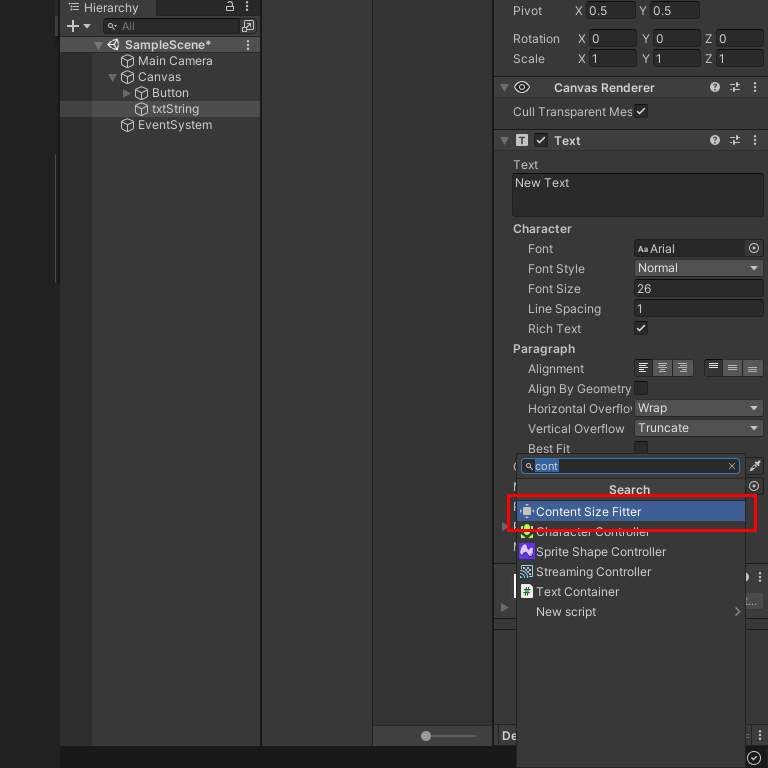
將 Horizontal Fit 設成 Preferred Size
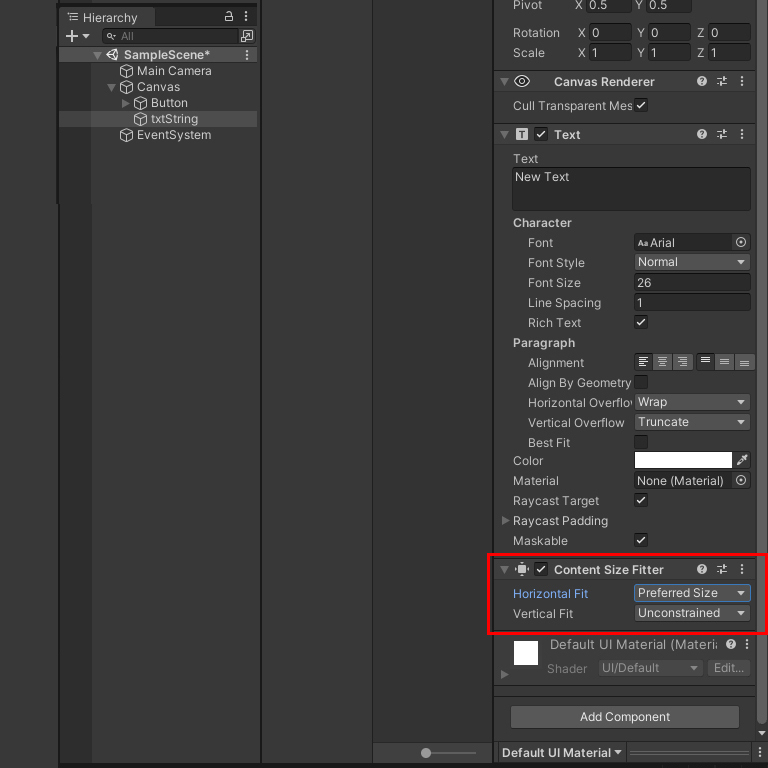
這樣就可以依據內容自動調整寬度了
預設 pivot X = 0.5 會讓字串從中間長
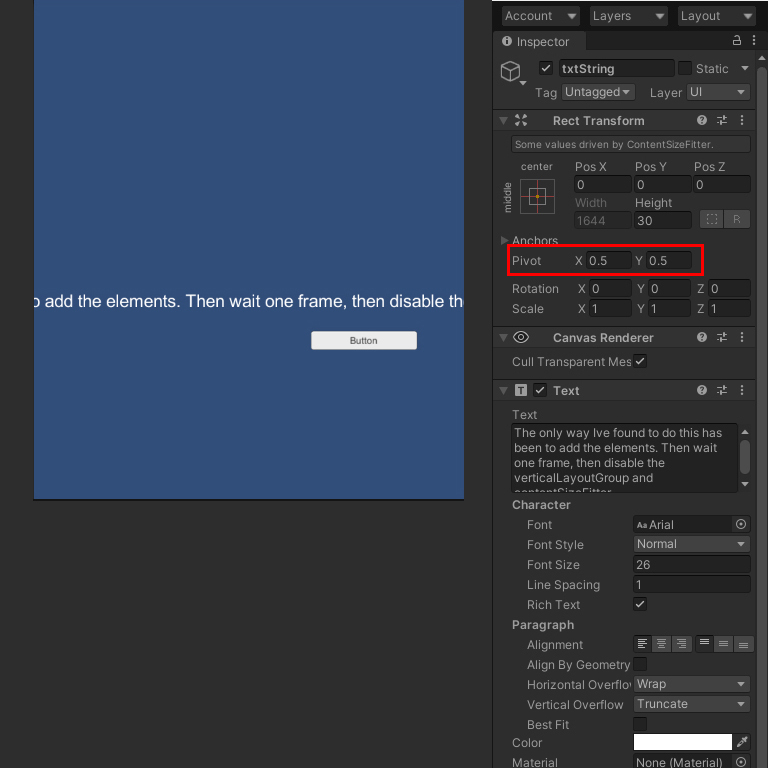
pivot X = 0 會讓字串往右邊長
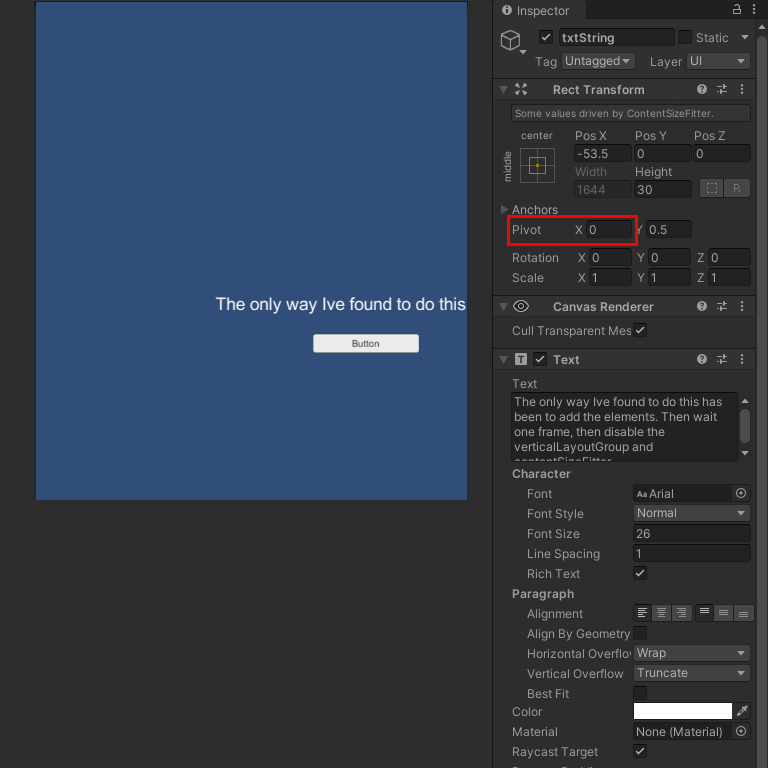
pivot X = 1 會讓字串往左邊長
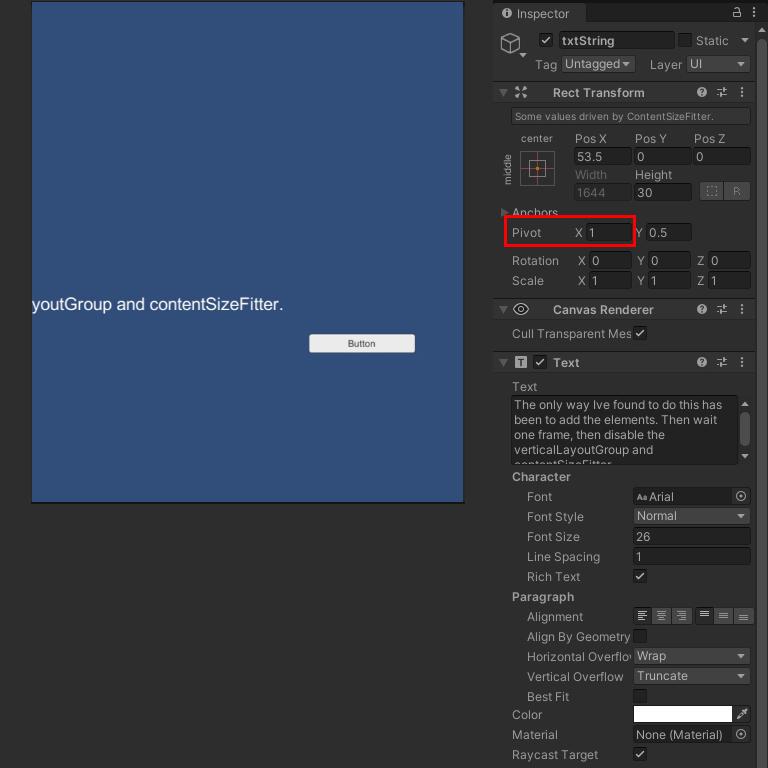
我們再把 pivot X 改回預設的 0.5
將 Content Size Fitter 改成這樣
執行後就會變成
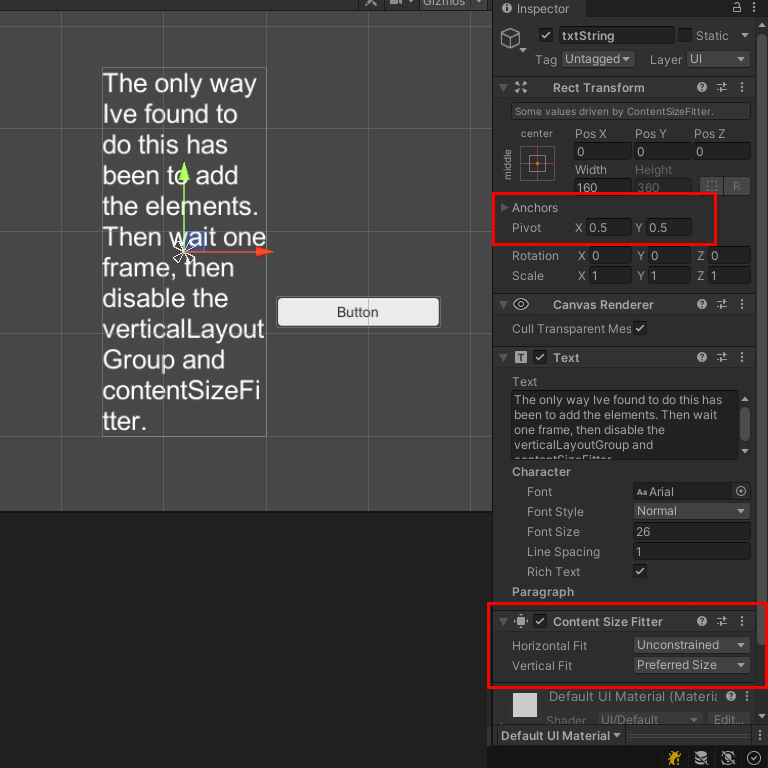
一樣修改 pivot Y 會從不同的方向長
pivot Y = 0
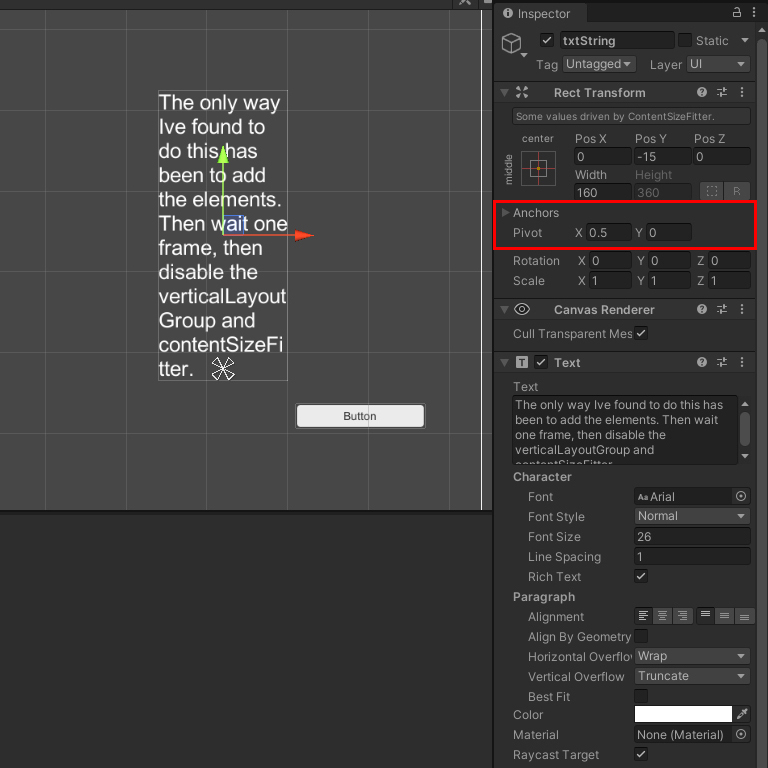
pivot Y = 1
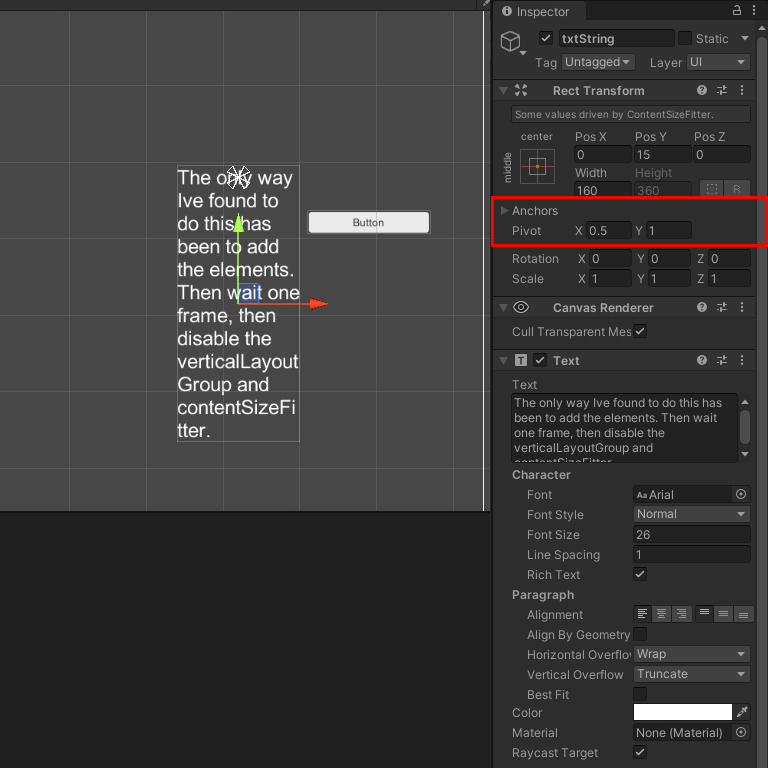
我們再把 pivot Y 改回預設的 0.5
來試試下面這段程式碼
測試程式碼3 ButtonChange.cs
public void OnButtonClick()
{
string str = "The only way Ive found to do this has been to add the elements. Then wait one frame, then disable the verticalLayoutGroup and contentSizeFitter.";
GameObject goText = GameObject.Find("txtString");
Text t = goText.GetComponent<Text>();
t.text = str;
RectTransform rcText = goText.GetComponent<RectTransform>();
Debug.Log("rcText.sizeDelta.y = " + rcText.sizeDelta.y.ToString());
}

會發現雖然設定了字串
但是尺寸並不是馬上改變的
而是在下一個 frame
如果要讓它馬上更改的話
就要增加一段
測試程式碼4 ButtonChange.cs
public void OnButtonClick()
{
string str = "The only way Ive found to do this has been to add the elements. Then wait one frame, then disable the verticalLayoutGroup and contentSizeFitter.";
GameObject goText = GameObject.Find("txtString");
Text t = goText.GetComponent<Text>();
t.text = str;
//即時更新尺寸
ContentSizeFitter sizeFitter = goText.GetComponent<ContentSizeFitter>();
sizeFitter.SetLayoutVertical();
RectTransform rcText = goText.GetComponent<RectTransform>();
Debug.Log("rcText.sizeDelta.y = " + rcText.sizeDelta.y.ToString());
}

延伸閱讀
Unity -UGUI Text 空格換行的問題
https://husking-studio.com/unity-ugui-text-02/